Journey to Inventing OmniCode: The Future of Programming
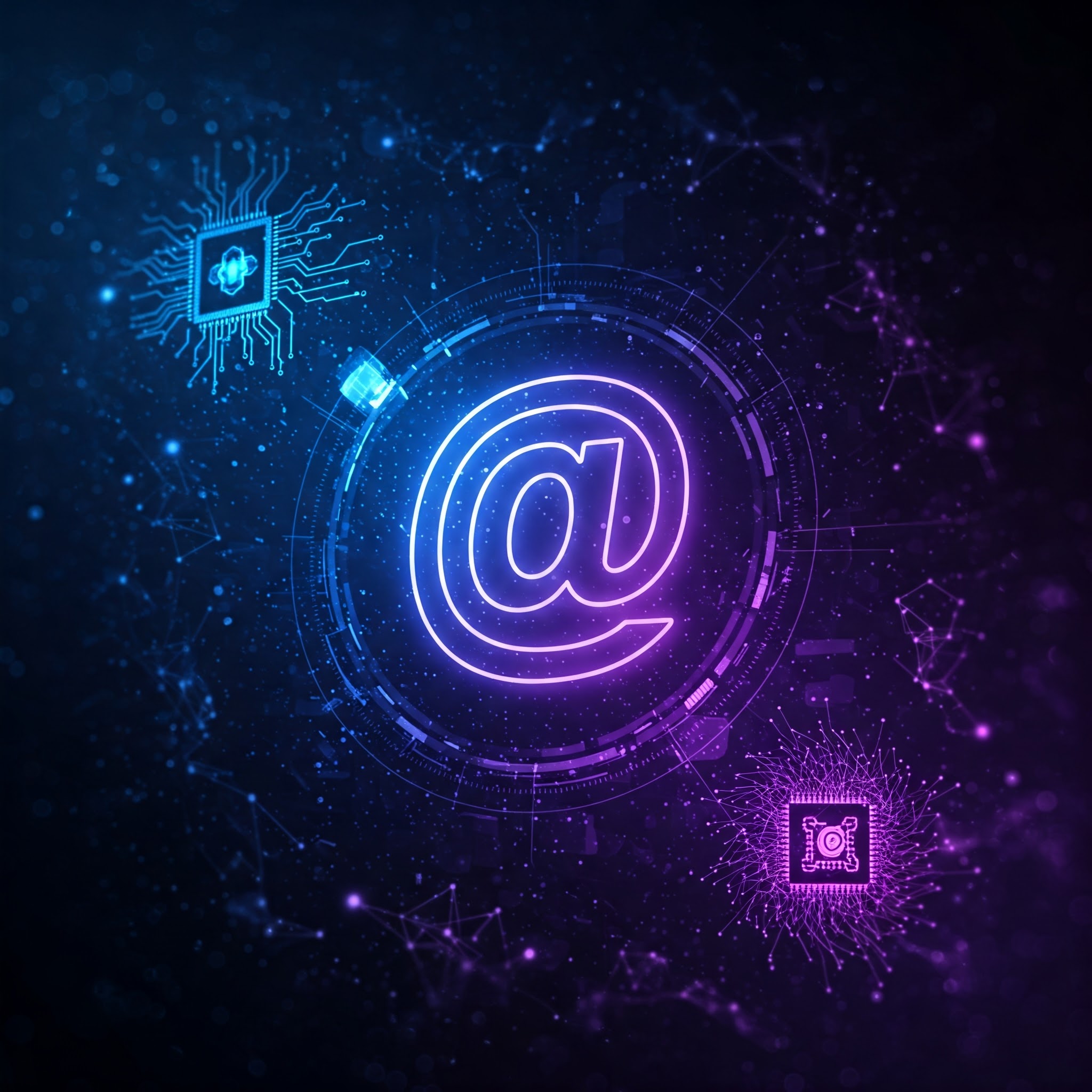
By Kevin Anjo
March 16, 2025
I’ve been coding for a while now, jumping between languages like Python, C, JavaScript, and even newer ones like Zig. They’re all great in their own ways—Python’s simplicity, C’s raw power, JavaScript’s flexibility—but lately, I’ve been feeling like something’s missing. Why do I need ten different tools to build one project? Why can’t I have a single language that does it all—backend, frontend, databases, GUIs, even hardware programming—without breaking a sweat? That’s when it hit me: I need to create my own programming language. I’m calling it OmniCode, and it’s going to be the most powerful, simplest, and most advanced language ever built.
The Spark: A Language That Does Everything
It started with a late-night thought: what if I could write one sheet of code that handles everything? I mean everything—a web app with a slick GUI, a backend server, a database that builds itself, and maybe even a little chipset tweak to blink an LED or run an AI model. No separate files, no juggling frameworks, just one clean, easy-to-read page. I imagined a language so versatile it could slip into an HTML file, a Python script, or a C program like it belonged there. And not just that—I wanted it to be powerful enough to program microchips, quantum computers, and AI systems, all while staying dead simple to use.
I’ve seen how fragmented coding can get. You’ve got React for frontend, Node.js for backend, SQL for databases, and then something like Rust or C if you want to touch hardware. It’s a mess! I want OmniCode to unify all that. One language, one syntax, one “sheet” of code—and it’s done. That’s my dream.
Dreaming Up the Features
So, I started sketching out what OmniCode would need. First, it has to be simple. I’m tired of languages that make you memorize a million keywords or wrestle with cryptic syntax. I want OmniCode to feel like writing a to-do list—intuitive, almost like talking to a friend. Something like “make a button” or “do a server” should just work. But simple doesn’t mean weak—it’s got to be powerful, too. I’m talking about handling quantum AI computations one minute and flipping a GPIO pin on a Raspberry Pi the next.
Next, it needs to be dynamic. I don’t want to manually set up databases or optimize performance—OmniCode should figure that out itself. Imagine writing a line like make database "users" { grow: auto }
, and boom, it creates a database that expands as you add data, no extra config needed. Or a server that spins up with one command and adapts to traffic spikes on its own. That’s the kind of magic I’m aiming for.
Then there’s versatility. I want OmniCode to run anywhere—on a web server, in a browser, inside a Python app, or even embedded in a Next.js project. I’m thinking of an @embed
tag that lets you drop it into any language, like a universal translator for code. And it’s not just about software—it should talk to hardware, too. I want to write a line like pin 13 -> high for 1s
and have it control a real chip, no fuss.
Finally, it’s got to be future-proof. Quantum computing’s coming, and AI’s already everywhere. OmniCode needs to handle both. I’m picturing a link ai "quantum_model"
command that seamlessly taps into whatever crazy tech we’ve got in 2030 or beyond. It’s not just a language for today—it’s for tomorrow.
My Plan to Build It
Turning this into reality won’t be easy, but I’ve got a rough roadmap. Step one is nailing the syntax. I’m leaning toward a mix of Python’s indentation and some natural-language vibes—short, clear commands like do
, make
, and link
. No semicolons or curly braces unless you want them. It’ll feel lightweight but scale up when you need it.
Then there’s the runtime. OmniCode needs a brain—a system that interprets the code, generates databases, optimizes performance, and transpiles it into other languages. I’m thinking it could compile to WebAssembly for web stuff, C for hardware, or even quantum assembly for the wild future stuff. That’s a big challenge, but I’ll start small—maybe a basic interpreter in Python or Rust to test the core ideas.
The “single-sheet” part is tricky. I want everything—GUI, logic, database—in one file, but it can’t get cluttered. My idea is to let the runtime figure out what’s what. If I write make gui { button "Click Me" }
, it knows that’s frontend. If I add make chipset "GPIO_1"
, it handles the hardware. Context is key, and the language should be smart enough to sort it out.
Embedding’s another hurdle. I’ll need a way to package OmniCode so it plays nice with HTML, PHP, React, you name it. Maybe a lightweight runtime library that other platforms can load, plus that @embed
tag to signal the switch. It’s got to feel seamless—like OmniCode was always meant to be there.
A Taste of OmniCode
Here’s a quick example I jotted down to see how it might look:
do app "MyApp" {
make gui {
button "Say Hi" -> trigger hello
}
do hello {
show "Hello, World!"
}
make database "logs" {
fields: { "message": text }
grow: auto
}
do server {
port: 3000
}
@embed "html" {
<p>Running OmniCode here!</p>
}
}
That’s it—one file that makes a web app with a button, a server, and a database that grows as you log stuff. Drop it in an HTML page, and it just works. That’s the vibe I’m chasing.
The Road Ahead
I know this is ambitious. People might say it’s impossible to pack all this into one language without it turning into a bloated mess. But I think it’s worth a shot. If I can pull it off, OmniCode could change how we code—making it faster, simpler, and more fun. I’ll start prototyping soon, maybe share some snippets online to get feedback. Who knows? Maybe in a few years, you’ll see OmniCode powering apps, chips, and quantum AIs all at once.
For now, it’s just me, my ideas, and a lot of coffee. But I’m excited. This isn’t just a language—it’s a revolution. What do you think? Want to join me on this ride?
Core Principles of OmniCode
- Unified Sheet: All code—logic, GUI, database, hardware interaction—lives in one file, with sections dynamically interpreted based on context.
- Dynamic Expansion: OmniCode adapts itself at runtime, generating databases, optimizing performance, or even writing subroutines as needed.
- Universal Embedding: It can run standalone or embed into other languages (e.g., HTML, Python, C) via a lightweight runtime or transpiler.
- Simple Syntax: Minimal keywords, intuitive structure, and self-explanatory commands, inspired by natural language and modern scripting.
- Infinite Power: Built-in support for high-level AI (quantum and classical), low-level chipset programming, and everything in between.
- Cross-Domain: Handles backend (servers, APIs), frontend (GUIs, animations), and hardware (chipsets, IoT) seamlessly.
Syntax Overview
- Keywords: Minimal and multi-purpose (e.g.,
do
,make
,link
,grow
). - Blocks: Defined by indentation (like Python) or curly braces
{}
for clarity. - Dynamic Typing: Variables adapt to context (e.g., a number can become a GUI button if used that way).
- Self-Contained: Databases, GUIs, and hardware specs emerge from the code itself—no external files needed.
- Embedding Tag: Use
@embed
to inject OmniCode into other languages.
Sample OmniCode Program
Let’s create a single-sheet program that:
- Builds a web app with a GUI (frontend).
- Sets up a backend API and auto-generates a database.
- Programs a chipset to blink an LED (hardware).
- Runs an AI model to predict something simple (e.g., weather).
- Embeds into a Python server.
Here’s what it might look like:
# OmniCode: WeatherBlink App
do app "WeatherBlink" {
# Frontend GUI
make gui {
button "Get Forecast" -> trigger predict_weather
label "Result" -> show weather_output
}
# Backend Logic + Auto-Generated Database
make database "weather_data" {
fields: { "date": text, "temp": number, "condition": text }
grow: auto # Dynamically expands as data comes in
}
do predict_weather {
link ai "quantum_model" { # Could be quantum or classical AI
input: current_date
output: weather_output = "Sunny, 25°C"
}
save weather_output to "weather_data"
return weather_output
}
# Hardware: Blink LED on chipset if sunny
make chipset "GPIO_1" {
if weather_output has "Sunny" {
pin 13 -> high for 1s, low for 1s, repeat 3
}
}
# Server Setup
do server {
port: 8080
route "/forecast" -> predict_weather
}
}
# Embed in Python (or HTML, C, etc.)
@embed "python" {
print("Starting OmniCode WeatherBlink...")
# OmniCode runtime auto-transpiles this into Python
}
How It Works
- GUI:
make gui
creates a cross-platform interface (web, desktop, or mobile) with a button and label. No separate HTML/CSS needed—it’s inferred. - Database:
make database
auto-generates a schema and storage system (e.g., in-memory or disk-based) that grows dynamically withgrow: auto
. - AI:
link ai
taps into a quantum or classical AI model (runtime decides based on hardware). It predicts weather here, but could do anything. - Hardware:
make chipset
interfaces with hardware (e.g., GPIO pins on a microcontroller) using simple commands. - Server:
do server
spins up a backend API accessible atlocalhost:8080/forecast
. - Embedding:
@embed "python"
lets this run inside a Python script, with the OmniCode runtime transpiling it to Python-compatible code. Swap “python” for “html”, “c”, or “zig”, and it adapts.
Key Features
- Simplicity: No boilerplate—just
do
,make
, andlink
get you started. Indentation keeps it clean. - Power: Handles GUI, backend, database, hardware, and AI in ~20 lines.
- Dynamic Expansion: The
grow
keyword lets the database evolve, and the runtime optimizes performance (e.g., caching, parallelizing). - Universal Compatibility: The
@embed
tag and runtime make it implantable anywhere—HTML, React, C, even quantum systems. - Chipset & Quantum Ready: Syntax supports low-level pin control and high-level AI model integration.
Why It’s the Most Powerful Language
- All-in-One: No need for separate tools (e.g., SQL for DB, JavaScript for frontend, C for hardware)—it’s all here.
- Self-Adapting: The
grow
andlink
features let it rewrite itself for efficiency or new tasks. - Cross-Platform: Runs on servers, browsers, chips, or quantum computers via a universal runtime.
- Beginner-Friendly: Reads like a to-do list but scales to expert-level complexity.